Programming - absolute basic
![]() |
fig: python logo |
Program is a set of instruction that makes computer usable. Imagine that you want to know the average speed you've reached during a long journey. You know the distance, you know the time, you need the speed.
Naturally, the computer will be able to compute this, but the computer is not aware of such things as distance, speed or time. Therefore, it is necessary to instruct the computer to:
- accept a number representing the distance;
- accept a number representing the travel time;
- divide the former value by the latter and store the result in the memory;
- display the result (representing the average speed) in a readable format.
Like we have our own mother language computer also do have their own language which is called machine language.
Language consists of following elements:
- An alphabets : a set of symbols used to build words of a certain language
- A lexis : (aka a dictionary) a set of words the language offers its users
- A Syntax: a set of rules (formal or informal, written or felt intuitively) used to determine if a certain string of words forms a valid sentence
- Semantics : a set of rules determining if a certain phrase makes sense
Programs should be correct in following sense:
- alphabetically - a program needs to be written in a recognizable script, such as Roman, Cyrillic, etc.
- lexically - each programming language has its dictionary and you need to master it; thankfully, it's much simpler and smaller than the dictionary of any natural language;
- syntactically - each language has its rules and they must be obeyed;
- semantically - the program has to make sense.
COMPILATION - the source program is translated once (however, this act must be repeated each time you modify the source code) by getting a file (e.g., an .exe file if the code is intended to be run under MS Windows) containing the machine code; now you can distribute the file worldwide; the program that performs this translation is called a compiler or translator;
INTERPRETATION - you (or any user of the code) can translate the source program each time it has to be run; the program performing this kind of transformation is called an interpreter, as it interprets the code every time it is intended to be executed; it also means that you cannot just distribute the source code as-is, because the end-user also needs the interpreter to execute it.
Due to some very fundamental reasons, a particular high-level programming language is designed to fall into one of these two categories.
There are very few languages that can be both compiled and interpreted. Usually, a programming language is projected with this factor in its constructors' minds - will it be compiled or interpreted?
First of all, the interpreter checks if all subsequent lines are alphabetically, lexically,syntactically and semantically correct or not . If the compiler finds an error, it finishes its work immediately. The only result in this case is an error message. The interpreter will inform you where the error is located and what caused it. However, these messages may be misleading, as the interpreter isn't able to follow your exact intentions, and may detect errors at some distance from their real causes.
Compilation vs. interpretation - advantages and disadvantages
|
Advantages | Disadvantages |
Compilation |
|
|
Interpretation |
|
|
Python is a widely-used, interpreted, object-oriented, and high-level programming language with dynamic semantics, used for general-purpose programming.
Guido van Rossum was implementing Python, he was also reading the published scripts from Monty Python’s Flying Circus.
Monty Python’s Flying Circus is a BBC Comedy TV series from the year 1969+. It is a highly viewed TV series and is rated 8.8 in IMDB.
One of the amazing features of Python is the fact that it is actually one person's work. Usually, new programming languages are developed and published by large companies employing lots of professionals, and due to copyright rules, it is very hard to name any of the people involved in the project. Python is an exception.
There are not many languages whose authors are known by name. Python was created by Guido van Rossum, born in 1956 in Haarlem, the Netherlands. Of course, Guido van Rossum did not develop and evolve all the Python components himself.
Python is very easy to learn, understand,install and deploy.
There are two main kinds of Python, called Python 2 and Python 3.
Linux users most probably have Python already installed.Documentation of python is excellent so it will be best if you go through documentation.
You can click here and Download python for the desire OS.
After installation to start your work, you need the following tools:
- an editor which will support you in writing the code (it should have some special features, not available in simple tools); this dedicated editor will give you more than the standard OS equipment;
- a console in which you can launch your newly written code and stop it forcibly when it gets out of control;
- a tool named a debugger, able to launch your code step by step and allowing you to inspect it at each moment of execution.
click on file tab and click on new to create new file and save the file. File will have extension as .py
You can write python code there .
You can simply do simple mathematical operation, You can print any thing by simply putting the word you want to print inside print function like if you write print("this will be printed") then the output will be this will be printed.It is this much simple.
You can simply write on the shell or you can write on new file to run you can simple press enter in shell and and select and f5 on file. you can also to terminal with path and run by typing python space filename.
Let's write the 1st code
print("Hello, World!")
As you can see, the first program consists of the following parts:
- the word
print
;
- an opening parenthesis;
- a quotation mark;
- a line of text:
Hello, World!
;
- another quotation mark;
- a closing parenthesis.
The word print that you can see here is a function name. That doesn't mean that wherever the word appears it is always a function name. The meaning of the word comes from the context in which the word has been used.
You've probably encountered the term function many times before, during math classes. You can probably also list several names of mathematical functions, like sine or log.
Python's functions, however, are more flexible, and can contain more content than their mathematical siblings.
A function (in this context) is a separate part of the computer code able to:
- cause some effect (e.g., send text to the terminal, create a file, draw an image, play a sound, etc.); this is something completely unheard of in the world of mathematics;
- evaluate a value or some values (e.g., the square root of a value or the length of a given text); this is what makes Python's functions the relatives of mathematical concepts.
Where do the functions come from?
- They may come from Python itself; the print function is one of this kind; such a function is an added value received together with Python and its environment (it is built-in); you don't have to do anything special (e.g., ask anyone for anything) if you want to make use of it;
- they may come from one or more of Python's add-ons named modules; some of the modules come with Python, others may require separate installation - whatever the case, they all need to be explicitly connected with your code (we'll show you how to do that soon);
- you can write them yourself, placing as many functions as you want and need inside your program to make it simpler, clearer and more elegant.
Of course, if you're going to make use of any already existing function, you have no influence on its name, but when you start writing your own functions, you should consider carefully your choice of names.
The only argument delivered to the
print()
function in the following example is a string:print("this is to be printed")
The print() function - the escape and newline characters
for example output for the following code
print("The itsy bitsy spider\nclimbed up the waterspout.")
print()
print("Down came the rain\nand washed the spider out.")
will be
The itsy bitsy spider
climbed up the waterspout.
Down came the rain
and washed the spider out.
The backslash (\
) has a very special meaning when used inside strings - this is called the escape characterfor more then one string argument
- a
print()
function invoked with more than one argument outputs them all on one line;
- the
print()
function puts a space between the outputted arguments on its own initiative.
Python offers another mechanism for the passing of arguments, which can be helpful when you want to convince the
print()
function to change its behavior a bit.The
print()
function has two keyword arguments that you can use for your purposes. The first of them is named end
.In order to use it, it is necessary to know some rules:
- a keyword argument consists of three elements: a keyword identifying the argument (
end
here); an equal sign (=
); and a value assigned to that argument;
- any keyword arguments have to be put after the last positional argument (this is very important)
sep
argument delivers the following results:print("My", "name", "is", "Jidesh", "baidya.", sep="-")
My-name-is-Jidesh-baidya.
like wise output for
print("My", "name", "is", sep="_", end="*")
print("jidesh", "baidya.", sep="*", end="*\n")
is
My_name_is*jidesh*baidya.*
apart from string we can also write
if we write
print('I\'m Jidesh Baidya.')
print("\\")
output will be I'm Jidesh Baidya
\
Literals are notations for representing some fixed values in code
What types of literals are the following four examples?"1.5", 2.0, 528, False
ans:
The first is a string, the second is a numerical literal (a float), the
third is a numerical literal (an integer), and the fourth is a boolean
literal.An operator is a symbol of the programming language, which is able to operate on the values.
+
, -
, *
, /
, //
, %
, ** are generally used operator in python
Different operator groups are as follows:
Arithmetic Operators
Assignment Operators
Comparison Operators
Logical operators
Identity operators
Membership operators
Bitwise Operators
Arithmetic operators:
Operator |
Name |
Description |
Example |
+ |
Addition |
Add 2
operands |
x+y |
- |
Subtraction |
Subtract right operand from left |
x-y |
* |
Multiplication |
Multiply
two operand |
X*y |
/ |
Division |
Divide left operand to right |
x/y |
% |
Modulus |
Reminder
of division |
x%y |
** |
Exponent |
Left operand raise to power of right |
X**y |
// |
Floor
Division |
Divide
the result into whole number |
x//y |
Arithmetic operators: division
A
/
(slash) sign is a divisional operator.The result produced by the division operator is always a float.
Arithmetic operators: exponentiation
A
**
(double asterisk) sign is an exponentiation (power) operator. Its left argument is the base, its right, the exponent.Arithmetic operators: integer division
A
//
(double slash) sign is an integer divisional operator. It differs from the standard /
operator in two details:Operators: remainder (modulo)
Comparison operators
Operator |
Name |
Description |
Example |
== |
Equal |
If the operands are equal than the condition becomes
true |
A==b |
!= |
Not equal |
If the two operands are not equal than
the condition becomes true |
A!=b |
> |
Greater than |
If left operand is greater than right operand then
the condition becomes true |
a>b |
< |
Less than |
If the left operand is less than
right operand then the condition becomes true |
A<b |
>= |
Greater than or equal to |
If left operand is greater or equal to right
operand then the condition becomes true |
A>=b |
<= |
Less than or equal to |
If the left operand is less than or equal
to right operand then the condition becomes true |
A<= |
Identity operators
- Identity operators are used to compare the objects
- Not if they are equal , but they are the same object with the same memory location
Operator |
Description |
Example |
is |
Returns
true if both the variabled are the same object |
A=1 A is b |
Is not |
Returns true if both variables are not
the same object |
A=[] A is not B |
Membership operators
- Used to test whether a value or variable is found in a sequence (String, list, tuple, set and dictionary)
Operator |
Description |
Example |
in |
Returns
true if a sequence with the specified value is present in the object |
X in y 2 in [1,2,3] returns true |
Not in |
Returns true if a sequence with the
specified value is not present in the object |
X not in y |
- Bitwise operators are used to compare (binary) numbers
Operator |
Description |
Name |
& |
Sets
each bit to 1 if both bits are 1 |
AND |
| |
Sets each bit to 1 if one of two bit
is 1 |
OR |
^ |
Sets bit to 1 if only one of the 2 bits is 1 |
XOR |
~ |
Invert all the bits |
NOT |
Operator |
Example |
Equivalent to |
= |
X=1 |
x=1 |
+= |
X+=5 |
x=x+5 |
-= |
x-=5 |
X=x-5 |
*= |
X*=5 |
X=X*5 |
/= |
x/=5 |
X=x/5 |
%= |
X%=5 |
X=x%5 |
//= |
x//=5 |
X=x//5 |
Operator |
Description |
() |
Parenthesis |
** |
Exponentiation |
+x,-x,~x |
Positive, negative, bitwise NOT |
*,@,/,//,% |
Multiplication, matrix, division, floor
division, remainder |
+,- |
Addition, Subtraction |
<<,>> |
Shifts |
& |
Bitwise AND |
^ |
Bitwise XOR |
| |
Bitwise OR |
In, not in, is, is
not, <,<=,>,>=,!,== |
Comparison, including membership
tests and identity test |
Not x |
Boolean NOT |
and |
Boolean AND |
or |
Boolean OR |
- The order in which an expression having multiple operator of same precedence is evaluated
- Almost all the operator have left-right associativity while the exponent "**' operator has right-left associativity
Keywords
set of predefined word is called keywords
you can use help("keywords") in interactive shell to see the list of key words
>>> help("keywords")
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
Identifiers in python
- Identifier in python is a name which can be used to identify a variable, function, class, module or other object
- Python is case sensitive programming language
- Rules to define a proper python identifiers
- Python identifier can be combination of lowercase/ uppercase letters, digits or an underscore
- A-Z and a-z both lower and upper case
- Digits 0-9
- Underscore_
- Identifier cannot begin with a digit
- we cannot use special characters (@,#,$,!,%,.) in the identifier name
- we cannot use keyword as a identifier
check validity of an identifier
import Keyword
keyword.isKeword("name")
or
"name".isidentifier()
Lines and indentation in python
no braces to indicate block of code for class, function or for flow control
block of code are denoted by line indentation
after if or for statement
in next line there is statement with 1 tab or 4 space
Comments
In python # is used for comment
"""
Triple quote
for
multiline
comment
"""
Python accept single('), double (") and triple (''' or """) quotes to denote string literals
Python statement is a each line in python script
Variable
In python variable are often special place to store a result. Variable may include number or value
If you want to give a name to a variable, you must follow some strict rules:
- the name of the variable must be composed of upper-case or lower-case letters, digits, and the character
_
(underscore)
- the name of the variable must begin with a letter;
- the underscore character is a letter;
- upper- and lower-case letters are treated as different (a little differently than in the real world - Alice and ALICE are the same first names, but in Python they are two different variable names, and consequently, two different variables);
- the name of the variable must not be any of Python's reserved words (the keywords - we'll explain more about this soon).
python function
function is the block of code which only runs when it is called
you can pass data known as parameters into a function.
a function can return data as result
return packet,brand //rutrun tuple
bring_milk(3,'sitaram')
bring_milk(brand="sitaram")
p,b=bring_milk(brand="Gayatri')
print(p,b) prints 1, Garatri
dynamic arguments
def bring-_milk((*args,**kargs)
print(args,"arguments")
print(kwargs,"keyword arguments")
bring_milk("ram',1,"hello",5.34,addreshh='lalitpur',roll=5)
bring_-mild(*tuple,**dist)
Python Data Types
Every object in python can be categorized into different types. The interpreter allocates memory based on data type of variable.
The data types in python are :
- Numbers
- Strings
- Boolean
- List
- Tuple
- Set
- Dictionary
Mutable and immutable objects
Mutable objects can be changed after it is created where as Immutable are the objects whose contents or states can't be changed.
Object types |
mutable |
Immutable |
int |
|
Yes |
Float |
|
Yes |
Bool |
|
Yes |
String |
|
Yes |
Tuple |
|
Yes |
List |
Yes |
|
set |
Yes |
|
dict |
Yes |
|
changing immutable object can be expensive as it creates a copy of itself
When to use?
Mutable
when there is a need to change the size or content of an object
Immutable
When the objects need to remain same or constant
Number
in number integer, float, and complex number are there.
Python list
is collection of different data types enclosed in big brackets([]).
slicing
[1:4] 1 to 3 index list data are displayed
there is negative indexing in python list as well
list operation
concatenation is done using + operator
methods in list
append
a=[1,2,3]
a.append(4)
extends
l1.extends(l2) =>l2 is added to l1
l1.insert(2,10)
it keep value of 10 in the second index of list l1
remove
is used to remove he value inside the parameter
pop(index)
is used to removed the index value
l1.index(number)
or l1.index(number,start,end)
gives number of index
l1.count(number)
count the occurrences of number in list
reverse
is used to reverse the element of list
l1=l2
this will be the same object
but if we use copy method like
l2= l1.copy()
l1 and l2 will be different object
we can know this by using id method on both list
List comprehension
provide a concise way to create lists
it consists of brackets containing an expression followed by a for clause, then zero or more for or if clauses
example:
l1=[x*5 for x in range(5)]
this results l1 to be [0,5,10,15,20]
this is equivalent to
l1=[]
for x in range(5):
l1.append(x*5)
this also result same
String
string in python are sequence of character data
python has a build in string class named "str"
string can be delimited using either single or double quotes or triple quotes
A valid string literal has same opening and closing delimiter
python does not have limit for characters in a string
String can also be empty
if we want to include a quote character as a part of string
- use alternate quote characters
- use escape character '\' (backslash)
Escape
Sequence |
“Escaped”
Interpretation |
|
\a |
ASCII bell
character |
|
\b |
ASCII
backspace character |
|
\n |
ASCII linefeed
character |
|
\r |
ASCII carriage
return character |
|
\t |
ASCII horizontal
tab(TAB) character |
|
\v |
ASCII vertical
t tab character |
|
\N{<name>} |
Character from Unicode
database with given <name> |
|
Raw string specifies that escape sequence in the associated string are not translated
r o R is added before beginning quote of the string literal to make it raw string
string can also be accessed like list and we can Iterate over string also
String Special Operators
if variable a hold 'Hello' and b holds 'World' then:
Operator |
Description |
Example |
+ |
Concate
or add 2 string |
a+b =>
HelloWorld |
* |
Multiple copy of same string |
B*2 => WorldWorld |
[] |
Slice
-give character from index |
a[4]
=> o |
[:] |
Range Slice- character from range |
a[2:5] will give llo |
In |
gives
True if character exist on string |
‘e’
in a => True |
Not in |
Gives true if character does not exist on string |
‘o’ not in a will return False |
Build in function that work on string
There are many python build in function that work on string and few of them are:
Function |
Description |
Len() |
Length
of string can be determined |
Bool() |
Returns Boolean value for an object |
Map() |
Apply
function on all the element of specified inerrable and return map object |
Print() |
Print data into console |
Slice() |
Return
slice object representing set of indices specified by range(star,stop,step) |
Type() |
Returns type if the object |
Python String methods
Methods |
Description |
capitalize() |
Capitalizes first
letter of string |
title() |
Returns
word that begin with UCase and rest are LCase |
upper() |
Returns uppercased
string |
lower() |
Returns
lowercased string |
split() |
Splits string
from left |
join() |
Merge
the string representation of elements in sequence seq into a string, with
separator string |
find() |
Returns the
index of first occurrence of substring |
Replace() |
Replace
Substring inside |
there are Multiple ways to format a string and few basic of them are:
- %formatting
- format() method
- formatted string literal (f-string)
- %(modulo) is known as interpolation operator
- % followed by the datatype that needs to be formatted or converted
- the % operation then substitutes the '%datatype' phrase with zero or more elements of the specified data type
%d |
Integer |
%s |
String |
%f |
Float |
%.2f |
Float with 2-digit
floating point |
%.4f |
Float with 4-digit floating point |
format() method
- Formatting can be handled by calling .format() on a string object
- General form of python .format() calls is as shown below:
- <template>.format(<positional_argument(s)>,<keyword_argument(s)>)
- In the <template> string replacement feilds are enclosed in curly braces ({})
- other text literal than the replacement fields are unchanged during the string formatting
>>'I am jon doe'
You must provide at least as many argument as there are replacement fields
if there are more arguments than the replacement the excessive arguments aren't used
you cannot mix manual field specification and automatic field numbering techniques together
formatted string literal(f-string)
- aka "formatted string literals", introduced in python 3.6
- f strings are string literals that have "f" at the beginning and curly braces containing expressions that will be replaced with their values
- example:
>>print(f"Hi! {name}")
Hi! John Doe
f-string are evaluated at runtime
What is Boolean data type?
- The boolean data type is ether true or False
- define by True and False keywords
- In numeric contexts (for example when used as the argument to an arithmetic operator), they behave like the integers 1 and 0 respectively
- The Boolean type is a subclass of the int class so that arithmetic using a Boolean still works
- Almost any value is evaluated to True if it has some sort of content
Python Tuples
- A tuple is a collection which is ordered and separated by commas
- Tuples are defined by enclosing the elements in parentheses (())
- In someway a tuple is similar to a list in terms of indexing, nested objects and repetition
- Unlike lists, tuples are immutable
- Tuple supports mixed datatypes
- tuple() function: a built-in function in Python that can be used to create a tuple
- Syntax: tuple (iterable)
- tuple() function accepts a single parameter iterable (optional)
- If an iterable is passed, the corresponding tuple is created
- If iterable is not passed, empty tuple is created
Tuple packing and unpacking
• we can create tuple without using parenthesis, that is called tuple packing.
>>>my tuple = 'John', 'Jane',24
>>> print (my _ tuple)
( 'John','Jane', 24)
• Tuple packing with only one element:
>>>my tuple = 'John', # you need a comma
>>print (my _ tuple)
( John' , )
The contrary is also possible, which is called tuple unpacking
my _ tuple = ( 'John', 'writer',23)
>>> name, profession, age = my _ tuple
print (name )
John
print (profession)
writer
print (age)
23
Number of variable on the left must match the number of values in the tuple
Accessing Tuple Elements
Indexing: we can use the index operator [ ] to access an item in a tuple, where the index starts from O.
Negative indexing is also possible in tuple
Slicing: We can access a range of items in tuple by using the slicing operator colon:
syntax: [start index (included): stop index (excluded): increment]
tuple can be deleted using del keyword
Tuple operation
- concatenation we can use + operator to combine tow tuples. This is called concatenation
- Repetition: we ca use * operator to repeat a tuple
- we can perform membership test using the in keyword
- we can iterate tuple
- Unlike Python lists, tuples does not have methods such as append(), remove(), extend(), insert() and pop() due to its immutable nature.
- There are only two tuple methods count() and index() that a tuple object can call.
Parameter: element - the element to be counted
index(): returns the index of the specified element in the tuple.
Syntax: tuple.index(element, start, end)
Parameters:
• element - the element to be searched
• start (optional) - start searching from this index
• end (optional) - search the element up to this index
index() method only returns the first occurrence of the matching element.
Some functions that can be used in tuples
sum(): Adds item of an iterable. While using sum() make sure tuple contains number data type only
ma(): returns the largest item
min(): returns the smallest value
sorted(): sort list of number in ascending order
reversed(): returns the reversed iterator of sequence
Dictionaries
- collection o key values pairs separated by comma(,)
- Each key value pair maps the key to its associated value
- Built in dictionary class named "dict"
- Insertion order of the item is preserved from python 3.7 and onwards
- In previous python version (before 3.7), dictionaries were un ordered collection
• Keys in a dictionary must be of immutable type(string, number, or tuple) and must be unique
• Values in a dictionary can be of any type and can repeat
• using built in dict() function
Accessing Values in Dictionary
• Not accessible using index value •
Value can be accessed using square brackets [ ]
• While trying to get value of non-existing key of dictionary, KeyError will be raised
• You can also assign the value to non-existing key in a dictionary
• Dictionary value is accessible using get () method on dictionary object
• Unlike square brackets, get ( ) method will not raise error while accessing non-existing key. It will return None instead.
• You can easily add default value, if None returned by get ( ) method.
Adding and Updating items in Dictionary
• Using key which is not already in use will create new key-value pair
•Using key which is already associated with some value will forgets the previous associated value
Removing Items from Dictionary
• pop ( ) method on a dictionary removes the item with provided key and returns value
•popitem ( ) method removes the item that was last inserted in dictionary and returns the removed pair (Changed in version 3.7: LIFO order is now guaranteed. In prior versions, popitem() would return an arbitrary key/value pair.)
• clear ( ) method is used to remove all key-value pair from the dictionary
• del keyword removes the entire dictionary itself
Restriction in Dictionary Keys and Values
• Restriction in dictionary keys:
• a given key can appear in a dictionary only once
• a dictionary key must be immutable
• Restriction in dictionary Values:
• there are no restrictions on dictionary values
• Note: Tuples can be used as keys, if they contain only immutable objects
• If a tuple contains any mutable object either directly or indirectly, it cannot be used as keys.
in operator is used to check dictionary membership . It checks key in dictionary
Build in function that works on Dictionary
There are many python build in function that work on dictionary and few of them are:
Function |
Description |
All() |
If all of the
dictionary are Ture ,return true |
Any() |
If any of the
dictionary are true return true, if empty return false |
Len() |
Return the length in
the dictionary |
Sorted() |
Return a new
sorted list of keys in dictionary |
Srt() |
Product a printable
string representation of a dictionary |
Type() |
Return type of
the object passed |
Dictionary Methods
Method |
Description |
Clear() |
Removes all elements from the
dictionary |
Copy() |
Returns a shallow copy of the dictionar |
Fromkeys(seq) |
Return a new dictionary with
keys from seq and values set to value |
Get(key,default=None) |
Return a value of the key. If the key does
not exist defaults to None |
Items() |
Return a list of the dictionary’s
items in (key,value) format |
Keys() |
Returns a list of the dictionary’s keys |
Pop(Key) |
Removes the item with the key
and return its value |
Popitem() |
Removes the item that was last inserted in
dictionary and return removed pair |
Setdefault(key,default=None) |
Like get(), but will set
dictionary key to default if key is not already in dictionary |
Update(dict2) |
Update the dictionary with the key/value
pairs from other, overwriting existing keys |
Values() |
Returns a list if dictionary’s
values |
Elegant way of creating new dictionary in Python
my_dict = {}
for i in range(5):
my_dict[i]= i**3
print(my_dict)
{0:0,1:1,2:8,3:27,4:64}
#using dictionary comprehension
my_dict = {a:a**3 for a in range(5)}
print(my_dict)
{0:0,1:1,2:8,3:27,4:64}
comprehension with condition are also possible
my_dict = {a:a**3 for a in range(5) i a%2 !=0}
print(my_dict)
{1:2,3;27}
Python Set
Set in Python
•Set is the collection of the unordered elements.
•They are represented by elements enclosed in the curly braces separated by comma.
•Set elements are unique.
•Set can also be created using set() built-in function.
•Set itself is mutable but the elements must be immutable.
•Example:
{1,2,3}
{"python", "is", "awesome"}
{1,2,3,(1,2,3)}
But if we try {[1, 2, 3], [4, 5, 6]} then the exception is raised
TypeError: unhashable type: 'list'.
Because, immutable elements are only allowed in the set, but list is mutable
Adding and Removing Set elements
• We can use add() or the update() method of the set class to add the elements.
• Example:
s1={1,2,3}
s1.add(4) results s1 to be {1,2,3,4}
s1.update([4,5,6]) results s1 to be {1,2,3,4,5,6}
•To remove the elements from the set we can use remove(), discard(), clear() or pop() method
Only difference between remove() and discard() is discard doesn't raise exception in case of absence of key whereas the remove() raise exception if the it is called with the value not present in the set.
clear() empty the set whereas pop() method randomly removes the element from the set. pop() doesn't take any argument if called with the set object.
Set Methods
Method |
Description |
Copy() |
Returns copy of the
set |
Difference() |
Returns the
difference of 2 or more sets as a new set |
Intersection() |
Returns the
intersection of two set as a new set |
Isdisjoin() |
Returns true
if two sets have a null intersection |
Issubset() |
Returns true if this
set contains another set |
Symmetric_diference() |
Returns the symmetric
difference of 2 sets as a new set |
Symmetric_difference_update() |
Updates a set with the
symmetric difference of itself and another |
Union() |
Returns the
union o sets in new set |
for the given sets A={1,2,3,4,5} and B=P{4,5,6,7,8} following operation can be performed
Operation |
Expression |
Result |
Union |
A|B or A.union(B) |
{1,2,3,4,5,6,7,8} |
Intersection |
A&B or
A.intersection(B) |
{4,5} |
Difference |
A-B or A.difference(B) |
{1,2,3} |
Symmetric Difference |
A^B or
A.symmetric_difference(B) |
{1,2,3,6,7,8} |
Frozenset
• Frozenset are the immutable sets.
• Normally the sets are mutable but the frozensets are immutable.
• They can be created using frozenset() function.
• The frozenset can also use the other set methods like copy(), union(), intersection() etc.
• add() and remove() methods can't be accessed by frozenset beacause of itsimmutable nature
• Example: A = frozenset([1, 2, 3, 4]) B = frozenset([3, 4, 5, 6])
Conditional Statement
• Conditional expression allows our program to make decisions.
• Every decision is taken with a Boolean expression which yields either true(l) or false (O) value.
• Conditional statements are handled by if statements in Python.
if statement
• Logical conditions such as equals (= not equals (!=), less than (<), greater than (>) and so on are used by if statements.
• If statements can further be implemented with else, elif and nested conditions.
• The simple example using conditional if statement to compare whether the value of variable satisfies the criteria or not.
if statement flow chart
if/else, if/elif/else statement
• We can easily create a chain of if statements.
• Once a condition satisfies the criteria then no other code blocks are executed. (in order from top to bottom)
• each statement is mutually exclusive which means it cannot occur at the same time.
• It is either condition1 or condition2 or condition 3 and so on.
• It is also possible to create non mutually exclusive statements.
• Here, condition 1 and condition 2 can satisfy at the same time.
• The else binds to the nearest if only. For example condition 3 if is bounded to the else condition.
ternary if statement
• We can simply create a one line if else statement.
• [on_true] if [condition] else [on_false]
Nested if statement
• Nested scenario occurs when an if else statement is present inside the body of another "if" or "else".
• It is better used to test a combination of conditions before making a proper decision.
Python Loops
Loops in Python world
• Loops help you to execute a block of code repeatedly
• Python world has two types of loops:
• For loop
• While loop
For loop
• For loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string).
• Syntax:
for var in sequence:
body Of loop
• var takes the value of the item inside the sequence on each iteration
• Loop continues through every item in the sequence until and unless we break it using the break keyword
For loop: the difference
• for statement in Python differs a bit from what we may be used to in C or Pascal.
• Unlike needing an arithmetic progression of numbers (as Pascal) or needing iteration step and halting condition (as C), for statement in python iterates over the items of any sequence.
flow Chart o the for loop:
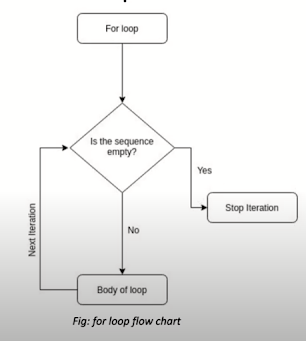
range() function:
• Built-in function used to generate a sequence of numbers.
• range() in Python(3.x) is just a renamed version of a function called xrange() in Python(2.x)
• Syntax:
range (start, stop, step size)
• start: integer starting from which the sequence of integers is to be returned
• stop: integer before which the sequence of integers is to be returned, range of integers end at stop — 1.
• step_size: integer value which determines the increment between each integer in the sequence
enumerate() function:
• Built-in function adds a counter to an iterable and returns it in a form of enumerate object
• allows you to loop over a collection of items while keeping track of the current item's index in a counter variable.
• Syntax: enumerate (iterable, start = 0)
• Iterable: any object that supports iteration
• start: the index value from which the counter is to be started, by default it is O
while loop (Indefinite Iteration)
• while loop executes a set of statements as long as a condition is true.
• Syntax:
while expression:
body of loop
• expression is condition that translates to either True or False
• body of loop contains the code block you want to execute if expression satisfies
Flowchart of while loop:
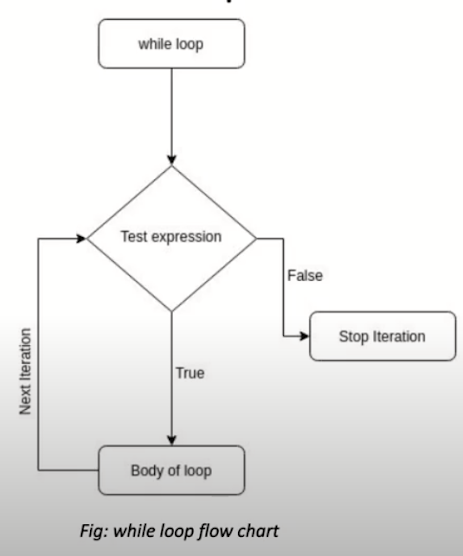
While loop examle:
sequence can also be used;
seq= [1,2,3,'loop','testing]
while seq:
seq.pop()
output
'testing'
'loop'
3
2
1
in each iteration an item is popped
in the last iteration the sequence is empty hence loop stops
Control statements
Used to alter the flow of code block in which it is being used
Supported control statements in Python are:
Break Statement
Continue Statement
Pass Statement
Break Statement
Used to terminate the loop or statement in which it is present
Syntax: break
After the execution of break statement, control is passed to the available code block In case of nested loops only the loops containing break statement will terminate
word="python"
for c in word:
if c.lower() in ['a','e','i','o','u']:
break
print(c)
P
Y
T
H
break statement flowchart
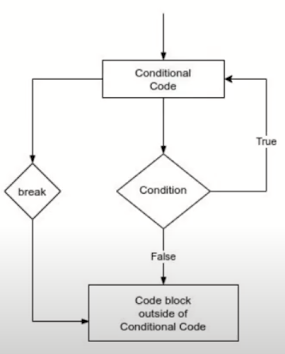
Continue Statement
Used to skip the current execution of loop or statement following the continue statement and the next iteration of the loop or statement will start
Syntax: continue
Forces to execute next iteration rather than terminating
to illustrate the continue statement lets investigate the following problem
you are told to scan each character of the word "PYTHON" and print it in the console. Later you are told not to print vowel letters in the word
word="python"
for c in word:
if c.lower() in ['a','e','i','o','u']:
continue
print(c)
P
Y
T
H
N
Pass Statement
• Placeholder for future code
• Used when statement is required syntactically but execution of the code is not wanted
•Like null operation (as nothing happens when executed, no errors will raise)
•Used to create empty control statement, function and classes.
• Syntax: pass
More on Control Statement
• else statement supported in python with a loop statement
• else statement is executed once the for loop gets exhausted iteration and in case of while loop, when the condition becomes false.
Iterator
Introduction (Iterator)
• In Python iterators are objects which can be iterated upon.
• We can define iterators as objects that can be used with 'for in loop'.
• Iterator follows the iterator protocol:
• Iterator must implement iter ( ) method
• Iterator must implement next ( ) method (we will cover these magic methods later)
Iterable
• Iterable objects in Python are objects from which we can get iterator.
• Strings, Lists, Tuples, Dictionaries, and Sets are the built-in iterable objects.
• All the iterable objects have iter ( ) method.
• We can use the function iter ( ) (which will call iter ( ) eventually) on iterable object to get an iterator.
Iterating through an Iterator
• next ( ) function is used on iterator (which will call next ( ) method eventually) to manually iterate through all the items of an iterator.
• Once all the items have been returned by next ( ) (no data for iteration), then it will raise Stoplteration.
How for loop works in Python? .
• At first, for loop creates an iterator object internally, by calling iter ( ) function on iterable.
• After that, the while loop repeatedly calls next ( ) function on iterator to retrieve values from it
• When the next ( ) function finishes returning items from iterator, it raises Stoplteration.
• After the raise of Stoplteration, the loop ends there.
Simple in iterator we can use loop.
Iterator are those object that can be changed into iterator
Generator in python
• Generator are simplified iterators (elegant kind of iterator)
• Generator allows you to write iterators in an elegant syntax, it avoid writing classes with iter ( ) and next ( ) methods.
• NOTE: Any generator also is an iterator(not vice versa)
Creating Generators in Python
• A generator can be created by defining a function which will use yield statement instead of return statement.
• yield statement also returns value but in a slightly different way than the return statement
• return statement terminates a function on one function call whereas yield statement keeps track of current states and resumes from the place where it left on successive function call.
• A generator function contains at least one yield statement and can have any number of other yield statements or return statements.
• Stoplteration is raised on further function call once the function terminates.
Creating Generators in Python
- we will started by creating a simplest infinite generator
while True:
yeild val
duplicates= duplcator(5)
print(type(duplicates))
<class 'generator'>
- next() unction works just like in the iterators form previous topics
def fibonacci_generator(max_value):
first,second,count=0,1,0
yeild first
yeild second
While True:
if count < max_value:
count +=1
first,second=second,first+second
yield second
else:
return
ficonacci_3= fibonacci_generator(3)
for fib in fibonacci_3:
print(fib)
Generator expression
• Generator expressions provide an effective shortcut for writing iterators.
• All generator topics covered till this slide were basically the generator functions.
• Generator expression adds another layer of syntactic sugar on top of generator function.
• Generator expressions generate values "just in time" like a class- based iterator or a generator function would, they are very memory efficient.
• Like the lambda function creates anonymous function, generator expression creates an anonymous generator function.
• In simple generator expression is a type of generator which is equivalent of list comprehension.
• A generator expression is like the list comprehension but, the square brackets are replaced by round parenthesis.
• Generator expressions are memory efficient than an equivalent list comprehension
greetings=('Namaste' for i in range(3))
print('type:',type(greetings),'\n)
print('obj',freetings,'\n)
print('messages: ')
for greet in greetings:
print(greet)
output
type: <class 'generator'>
obj : <generator object <genexpr> at 0x7f4cf4032cf0>
message:
Namaste
Namaste
Namaste
# generator expression template:
gen_exp = (expression for item in collection if condition)
# generator function template:
def my _ generator( ) :
for item in collection:
if condition:
yield expression
If a generator expression is used as the single argument to a function, then the parenthesis surrounding a generator expression can be ignored.
Good practice on writing beautiful generator expression
• There is no specific rule to follow how deep your generator expression can be nested.
• We would recommend generator expression that is not more than two levels of nesting.
• It would be easy to maintain in the future
• It would be easy to understand what we are going to do with the generator expression
• For complex iterators, it is better to write a generator function or even a class-based iterator chains.
• We will cover chaining of generator in next slide.
Chain of generators
• Chaining together multiple generators
• Generators can be chained together to form highly efficient and maintainable data processing pipelines.
• We chain multiple generators in a sequence so that the yielded result of a generator is passed to another generator for processing.
• Inside chaining of generators, the data processing happens one element at a time.
we define 3 generator function to illustrate the example
Function in python
def integers( ) :
for i in range(1,5)
yield i
integers=range(1,5)
def squared( seq):
for t in seq:
yield i*i
squared=(i*i for i in integers)
def negated( seq):
for in seq:
yield -i
negated=(-i for i in squared)
Is generator expression better than generator function in generator chaining?
• Generator expression cannot be configured with function arguments
• We can't reuse the same generator expression multiple times in the same processing pipeline.
• We can use the mix of generator expression and generator function
Iterators, Iterables, Generators in nutshell
Function in Python
- Block of organized, reusable code that is used to perform a single, related action
- High degree of code reusing
- Easy to break down the large programs into smaller and modular chunks
- Easy to organize and manage larger programs
- Types of functions in Python:
- Built-in functions
- User-defined functions
- There are many built-in functions in Python, and we can create our own custom functions
syntax for defining function is as follows:
def <function_name>([<parameters>]):
<Statement(s)>
Component |
Meaning |
Def |
Informs
python that function is being defined |
<Function_name> |
A valid python identifier that names the function |
<parameters> |
An
optional comma separated list of parameters that may be passed to function |
: |
Punctuation that denotes end of python function header
(name and parameter) |
<statements(s)> |
A
block o valid python statements |
Just like with other python object you can store function in a data structure
Map, filter and reduce function in python
• lambda function is a small anonymous function
• Uses lambda keyword
• Anonymous functions are defined without a name
• Syntax: lambda arguments: expression
• Lambda functions can have any number of arguments, but are syntactically restricted to a single expression
• Rules which need to be considered while defining a function:
• Function block starts with keyword 'def' followed by the function name and parenthesis ( ( ) ). (function naming follows the rule of identifier naming, explained in the python basics )
• Parameters(arguments) are optional and should be placed within the parenthesis if used.
• Code block within every function starts with colon (:) and block itself is indented.
• Docstring of a function is optional
• The return statement is optional
eg.
sqr=lambda x: x**1
print(sqr(4)
16
it is same as
def sqr(x):
return(x**2)
print(sqr(2))
16
(lambda x: x 6 >>> add = lambda a, add (2, 5) >>> mul lambda a, b (2, 16) 32 lambda a, b, 17 c c
Difference between function and lambda:
>>> add = lambda x, y: x + y
>>> add
<function < lambda> at Ox7ff63d9c5400>
>>>def add (x, y):
return x + Y
>>> add < function add at Ox7ff63d9c5488>
• Both functions add two integers, accept two arguments
• But you may notice that the naming is different
• The function name is add for a function defined with def, whereas the Python
Difference between function and lambda:
Python lambda I filter, map, reduce
• filter(): built-in function that takes in a function and a list as arguments
• It takes a predicate as a first argument and an iterable as a second argument.
• The function is called with all the items in the list and a new list is returned which contains items for which the function evaluates to True.
even= lambda x: x%2 ==0
list(filter(even,[1,2,3,4,5,6,7,8]))
[2,4,6,8]
• It takes a predicate as a first argument and an iterable as a second argument.
• New list is returned which contains all the lambda modified items returned by that function for each item
>>>sqr= lambda x: x* *2
>>>list( (map (sqr, [1, 2, 3, 4,5,6,7,8)
[1,4,9,16,25,36,49,64]
Example:
map (lambda x: x* 2, range (1, 4) )
>>>print (x)
<map object at Ox7ff63d9c0f60>
>>>print (list (x) )
[2,4,6]
• Function above uses map function and lambda, which maps values from range function (start =1, stop = 4-1) to the lambda function which double the value (x* 2) • If we print variable x, it shows map object because map() function returns map object, we convert it to a list in the final step
• We can write it in a single step as: list (map (lambda X : X* 2))
Python lambda I filter, map, reduce
• reduce(): function that takes in a function and a list as arguments, this is a part of functools module
• It is called with a lambda function and a list and a new reduced result is returned • This performs a repetitive operation over the pairs of the list
>>>from functools import reduce
>>>add= lambda x, y: x+y
>>>reduce (add, [1, 2, 3, 4, 5, 6])
21
• Here the results of previous two elements are added to the next element and this goes on till the end of the list like (((((1+2)+3)+4)+5)+6).
filter ===>filter out one element and return iterator of the meet condition
Python decorators
What are python decorators?
• A decorator is a design pattern in Python that allows a user to add new functionality to an existing object without modifying its structure
• decorators add functionality to an existing code, which is also called metaprogramming as a part of the program tries to modify another part of the program at compile time
• Decorators are usually called before the definition of a function you want to decorate.
Prerequisites for learning decorators:
• Functions
• Higher-order functions
• closures in Python
• We have already discussed about functions and higher order functions and now we'll cover closures
What are python closures?
The greet() function is called with "Hello world" as argument and the returned function is bound to the name message.
Closure is a function (object) that remembers its creation environment (enclosing scope). In this case, "Hello world" gets attached to the code. This is called Python closure
Python closure criteria:
• There must be a nested function
• The nested function must refer to a value defined in the enclosing function
• The enclosing function must return the nested function
Decorator:breakdowm
• We have a regular function ordinary() which prints 'ordinary!' When called
• We have a decorator function which has an inner function inner func that calls the function passed as argument in decorate_me
• decorate_me() returns inner_func, as discussed in closure.
Decorator:
breakdown
>>>ordinary( )
ordinary!
>>> decorated = decorate_me(ordinary)
# decorating the ordinary function
decorated( )
It is now decorated!
ordinary!
• Here, decorate_me() is a decorator, function ordinary() got decorated and the returned function was given the name decorated.
• decorate_me(ordinary) returns a function that we call on the final step to see the decorated result.
• We can see that the decorator function added some new functionality to original function
Decorator: breakdown
• Generally, we decorate a function and reassign it as, ordinary = decorate_me(ordinary)
• So that when you call ordinary() it is decorated
• This is a common construct and for this reason, Python has a syntax to simplify this.
• We can use the @ symbol along with the name of the decorator function
Chaining decorators:
• We can decorate a function multiple times by same decorator or multiple decorators.
• Let's create another decorator add info, which adds info as shown.
• Here, the function shout() has been decorated multiple times
What is Object Oriented Programming(OOP)?
• A programming paradigm which provides a means of structuring programs so that properties and behaviors are bundled into individual objects.
• It allows us to think of the data in our program in terms of real- world objects, with both properties and behaviors.
• Properties define the state of the object. This is the data that the object stores.
• Behaviors are the action take. Oftentimes, this involves using or modifies of our object.
Classes
• A class is a blueprint for the object
• It is a user-defined prototype for an object that defines a set of attributes that characterize any object of the class.
Classes: Creating Classes
• Start with the class keyword to indicate that you are creating a class
• The keyword is followed by the name of the class.
• According to the PEP 8 style guide, class names should normally use the CapWords convention I.e., CamelCase notation.
• Note: When using acronyms in CapWords, capitalize all the letters of the acronym. Thus HTTPServerError is better than HttpServerError.
Classes:
Creating Classes
class Vehicle:
pass
• We have used keyword class to indicate that we are creating a class.
• We have used keyword pass in the place where the code will eventually go
• This creates a class named Vehicle, this process is called class definition
Objects
• An object (instance) is an instantiation of a class.
• Class definition defines the description for the object and hence no memory or storage is allocated
• Once we have our class, we can instantiate it to create a new object from that class.
• The created object has the type of the class it was instantiated from.
Instantiating classes:
(creating objects)
obj = Vehicle( )
• Here, obj is an object of class Vehicle
Class and Instance Attributes
• Classes contain characteristics called Attributes: instance attributes and class attributes.
• Instance attribute: Instance Attributes are unique to each object, (an instance is another name for an object).
class Vehicle:
def color,(self,color,doors):
self.color= color
self.doors = doors
• Here, any Vehicle object we create will be able to store its color and door. We can change either vehicle object, without affecting any other objects we've created
Class and Instance Attributes
__ init__():__ init__ is called the initializer.
• It is automatically called when we instantiate the class.
• Its job is to make sure the Class has any attributes it needs.
• It also makes sure that the Object is in a valid State When it'S instantiated, it'd be invalid if user try instantiating an Object using negative value for doors attribute, wouldn't it?
Class and Instance Attributes
class attributes are unique to each class.
Each instance of the class will have this attribute
class Vechicle:
category='car'
def __init__(self,color,doors):
self.color=color
self.doors=doors
here category is a class attribute
class and Instance Attributes: (Example)
• A class is an example of encapsulation as it encapsulates all the data that is member functions, variables, etc.
Polymorphism in Python:
• Polymorphism is derived from Greek words:
Poly :many
morphism forms
• Same method is defined on object of different types.
Two child class Bike and Car are inherited from parent class Vehicle
class Vehicle:
def __init__(self,model):
self.model = model
def info(self):
return f'A vehicle info.'
def max_speed(self):
return 140
class Bike(Vehicle):
def max_speed(self):
return 130
class Car(Vehicle):
def max_speed(self):
return 120
• From above example, while trying to access the same method info() and from objects of different classes we will get the
following result:
• Info() method will return the same result as we initialized
• method will return the value returned from object specific class.
Method Overloading:
Method Overriding:
def info(self):
• Define any of these methods and an object is considered a descriptor and can override default behavior upon being looked up as an attribute.
else Clause
Understanding try....except....else....finally block
Binary and Text Mode
Thank you for sharing wonderful information with us to get some idea about that content.
ReplyDeleteBest Python Training Online
Python Training Online Courses